Simplifying Android Testing with Compose
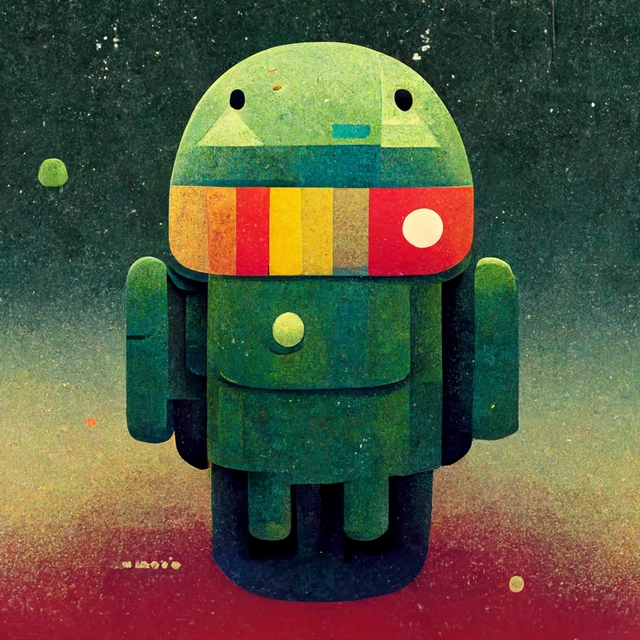
Jetpack Compose is a modern toolkit for building native Android UIs. It allows developers to create UIs using a declarative syntax, making it easier to build and maintain complex user interfaces. Compose was introduced in 2020 and has since gained popularity due to its simplicity and efficiency.
Compose simplifies Android testing by providing a more efficient and streamlined process. With Compose, developers can test individual components of their UI in isolation, rather than testing the entire UI at once. This makes it easier to identify and fix issues in specific areas of the UI, improving the overall quality of the application.
To get started with Compose testing, you'll need to add the Compose UI testing library to your project's dependencies. You can do this by adding the following line to your app's build.gradle file:
Next, you can create a test for a Compose UI component by using the createComposeRule() function to set up a Compose testing environment. For example, to test a TextField component, you might write the following test:
1class TextFieldTest {23@get:Rule4val composeTestRule = createComposeRule()56@Test7fun textFieldTest() {8composeTestRule.setContent {9TextField(value = "Hello, World!", onValueChange = {})10}1112val textField = composeTestRule.onNodeWithText("Hello, World!")13textField.assertExists()14textField.performClick()15}16}
In this test, we use the setContent() function to set the content of the Compose testing environment to a TextField component. We then use the onNodeWithText() function to locate the TextField element and perform assertions on its state.
Here are some tips for writing Compose tests:
- Use the function to set up a Compose testing environment.
- Use the function to set the content of the Compose testing environment.
- Use the function to locate UI elements and perform assertions on their state.
- Use the function to simulate user interactions with UI elements.
- Test individual components in isolation, rather than testing the entire UI at once.
Jetpack Compose provides a more efficient and streamlined approach to Android testing. With Compose, developers can test individual components of their UI in isolation, making it easier to identify and fix issues. Compose also provides a set of testing utilities that make it easy to write tests for UI components. By following these tips, you can start writing efficient and effective tests for your Compose UI components today.